/* ********************************************************************
* Create a new scrollObject:
*
* var scroll1 = new scrollObject("scr1", 120, 120, "up", 5000, 1.4);
*
* The arguments for this object are as follows:
* a. - ID of the target tag (from step 1)
* b. - Width (in pixels) of your scroller
* c. - Height (in pixels) of your scroller
* d. - Scroll direction: one of "up", "down", "left" or "right"
* e. - Amount of time to pause before next scroll begins (ms)
* f. - Slide-in speed of your scroller (1.001 up to width or height)
*
*************************************************** BEGIN CODE ***** */
// ***** Sample scroller #1
var scroll1 = new scrollObject("scr1", "249", 40, "left", 5000, 1.15);
var scroll2 = new scrollObject("scr2", 180, 139, "up", 5000, 1.15);
scroll1.block[0] = '[2008-06-25] Dubai --- Egypt';scroll2.block[0] = '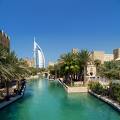
Dubai --- Egypt';
/* ********************************************************************
* The Mighty ScrollObject
* - Don't edit this if you know what's good for ya!
*
*/
function scrollObject(main, width, height, direct, pause, speed) {
var self = this;
this.main = main;
this.width = width;
this.height = height;
this.direct = direct;
this.pause = pause;
this.block = new Array();
this.offset = (direct == "up" || direct == "down") ? height : width;
this.speed = Math.max(1.001, Math.min(this.offset, speed));
this.blockprev = 0;
this.blockcurr = 1;
this.motion = false;
this.mouse = false;
this.scroll = function() {
if (!document.getElementById) return false;
this.main = document.getElementById(this.main);
while (this.main.firstChild) this.main.removeChild(this.main.firstChild);
for (var x = 0; x < this.block.length; x++) {
var table = document.createElement('table');
table.style.position = "absolute";
table.style.width = this.width + "px";
table.style.height = this.height + "px";
table.style.overflow = "hidden";
table.cellPadding = table.cellSpacing = table.border = "0";
table.style.left = table.style.top = "0px";
if (x) {
switch (this.direct) {
case "up": table.style.top = this.height + "px"; break;
case "down": table.style.top = -this.height + "px"; break;
case "left": table.style.left = this.width + "px"; break;
case "right": table.style.left = -this.width + "px"; break;
}
}
var tbody = document.createElement('tbody');
var tr = document.createElement('tr');
var td = document.createElement('td');
td.innerHTML = this.block[x];
tr.appendChild(td);
tbody.appendChild(tr);
table.appendChild(tbody);
this.main.appendChild(this.block[x] = table);
}
this.main.style.position = "relative";
this.main.style.width = this.width + "px";
this.main.style.height = this.height + "px";
this.main.style.overflow = "hidden";
if (this.block.length > 1) {
this.main.onmouseover = function() { self.mouse = true; }
this.main.onmouseout = function() { self.mouse = false; }
setInterval(function() { self.scrollLoop(); }, this.pause);
}
}
this.scrollLoop = function() {
if (!this.motion && this.mouse) return false;
if (!(this.offset = Math.floor(this.offset / this.speed))) {
var blocknext = (this.blockcurr + 1 >= this.block.length) ? 0 : this.blockcurr + 1;
} else this.motion = true;
switch (this.direct) {
case "up":
this.block[this.blockcurr].style.top = this.offset + "px";
this.block[this.blockprev].style.top = (this.offset - this.height) + "px";
if (!this.offset) this.block[blocknext].style.top = this.height + "px";
break;
case "down":
this.block[this.blockcurr].style.top = -this.offset + "px";
this.block[this.blockprev].style.top = (this.height - this.offset) + "px";
if (!this.offset) this.block[blocknext].style.top = -this.height + "px";
break;
case "left":
this.block[this.blockcurr].style.left = this.offset + "px";
this.block[this.blockprev].style.left = (this.offset - this.width) + "px";
if (!this.offset) this.block[blocknext].style.left = this.width + "px";
break;
case "right":
this.block[this.blockcurr].style.left = -this.offset + "px";
this.block[this.blockprev].style.left = (this.width - this.offset) + "px";
if (!this.offset) this.block[blocknext].style.left = -this.width + "px";
break;
}
if (!this.offset) {
this.motion = false;
this.blockprev = this.blockcurr;
this.blockcurr = blocknext;
this.offset = (this.direct == "up" || this.direct == "down") ? this.height : this.width;
} else setTimeout(function() { self.scrollLoop(); }, 30);
}
}